In this post I will going to show you, how to extend the validateField method on a table. As example I used the SalesLine, where I will create a post handler to extend the validateField method.
This kind of extensions were already there to be used in AX 2012, but since we have to work only with extensions now, events got a lot more focus nowadays.
Let’s go: ValidateField Extension
First search the SalesLine table in the AOT, then right click the table and select “Open designer”.
Go to the methods node, to the validateField method, right click, “Copy event handler method” and select “Post-event handler”.
Now create a new class and paste the copied event handler into it
class SaleLine_Events
{
[PostHandlerFor(tableStr(SalesLine),tableMethodStr(SalesLine, validateField))]
public static void SalesLine_Post_validateField(XppPrePostArgs args)
{
SalesLine salesLine = args.getThis();
FieldId fieldId = args.getArg("_fieldId");
boolean ret = args.getReturnValue();
switch(fieldId)
{
case fieldNum(SalesLine,LinePercent):
if (salesLine.LinePercent > 10)
{
ret = ret && checkFailed("Line per cent is to high!");
}
break;
}
args.setReturnValue(ret);
}
}
Here I set a few new variables. First of all I called the getThis() method on the passed argsargument.
The actual field to validate is more than interesting for us, for this reason we need to get the passed argument from the original method. In the AOT find the SalesLine again, right click it and select “View code”.
Search for the validateField method and you get the original argument’s name.
public boolean validateField(FieldId _fieldId)
With getArg(“_fieldId”) the original value will be retrieved. To decide if a field is valid or not, the original boolean value has to be known (getReturnValue()) and also a new return value has to be set (setReturnValue(ret)), with our own validation.
Result
In the example above the per cent per line is checked, if it is greater than 10, it will return false and post a message to the infolog.
Here I entered 11.
|
Pavan Ax Technical
Wednesday, January 30, 2019
D365 Extensions: Extend the validateField Method on a Table
Thursday, January 24, 2019
Show the table browser in D365
I just wanted to share this tip I have picked up from Marc Hugelshofer in a forum.
In your browser you can open the table browser by entering an URL that looks something like this:
https://(the url of your ax instance)/Default.htm?mi=SysTableBrowser&prt=initial&cmp=USMF&tablename=CustGroup&limitednav=true
In your browser you can open the table browser by entering an URL that looks something like this:
https://(the url of your ax instance)/Default.htm?mi=SysTableBrowser&prt=initial&cmp=USMF&tablename=CustGroup&limitednav=true
the "prt=initial" and "limitednav=true" are optional and can probably be left out. So for example:
https://(the url of your ax instance)/Default.htm?mi=SysTableBrowser&cmp=USMF&tablename=CustGroup
https://(the url of your ax instance)/Default.htm?mi=SysTableBrowser&cmp=USMF&tablename=CustGroup
prt is for (deprecated) partition functionality, and limitednav gets rid of filters and the navigation pane (which you might want, but probably not). On the other hand, you can also add lng=[language] to the URL if needed.
Wednesday, January 23, 2019
orig() method in AX
Hi All,
There is a method named orig() in AX which refers to the last saved state of the current table buffer. The SalesTable form uses orig() method in lot of places in its datasource methods.You could find the code in the write method of the SalesTable datasource.
In the SalesLine DataSource under the SalesQty field you could find the following code in the modified() method.
if (salesLine.orig().SalesPrice!= salesLine.SalesPrice)
{
//some code here
}
In this case salesLine.orig().SalesPrice is the original (already saved)
price, while salesLine.salesPrice is the price that the user is attempting to modify.
There is a method named orig() in AX which refers to the last saved state of the current table buffer. The SalesTable form uses orig() method in lot of places in its datasource methods.You could find the code in the write method of the SalesTable datasource.
In the SalesLine DataSource under the SalesQty field you could find the following code in the modified() method.
if (salesLine.orig().SalesPrice!= salesLine.SalesPrice)
{
//some code here
}
In this case salesLine.orig().SalesPrice is the original (already saved)
price, while salesLine.salesPrice is the price that the user is attempting to modify.
Monday, January 21, 2019
Understanding: Models and Model Store [AX2012]
The very first question in my mind is the difference between Model and Model Store in AX 2012 while i have started to exploring AX 2012.
I found the no. of posting related to this from different materials but below is clearly understood explanations.
Basic Info:
Models were introduced in Microsoft Dynamics AX to help partners and customers more easily install and maintain multiple solutions side by side in the same layer. This topic introduces the concept of models, and describes how models relate to layers and label files. This topic also describes the model store, which is the part of the Microsoft Dynamics AX database in which models are stored.
Models:
A model is a set of elements in a given layer. Each layer consists of one or more models. Each layer contains one system-generated model that is specific to that layer. Every element in a layer belongs to only one model. In other words, no element can belong to two models in the same layer, and every element must belong to a model.
I found the no. of posting related to this from different materials but below is clearly understood explanations.
Basic Info:
Models were introduced in Microsoft Dynamics AX to help partners and customers more easily install and maintain multiple solutions side by side in the same layer. This topic introduces the concept of models, and describes how models relate to layers and label files. This topic also describes the model store, which is the part of the Microsoft Dynamics AX database in which models are stored.
Models:
A model is a set of elements in a given layer. Each layer consists of one or more models. Each layer contains one system-generated model that is specific to that layer. Every element in a layer belongs to only one model. In other words, no element can belong to two models in the same layer, and every element must belong to a model.
A model is permanently associated with the layer that is created in. If you need to move one of your models from one layer to another, you must create a project from the model in the AOT, export the project as an xpo file, create a target model in the desired layer, delete the original model to avoid having to resolve layer conflicts, and import the xpo file to the target model. If you are moving elements between models in the same layer, you can use the Move to model command in the AOT.
ModelStore:
Models are stored in the model store. The model store is the part of the Microsoft Dynamics AX database in which all application elements for Microsoft Dynamics AX are stored. Customizations are also stored in the model store. The model store replaces the Application Object Data (AOD) files that were used in earlier versions of Microsoft Dynamics AX. Models that have been installed in the model store are used at run time.
Note: Models can be exported to files that have the .axmodel extension. These files are called model files. Model files are deployment artifacts. Model files can be signed with strong name signing and Microsoft Authenticode signing.
How to manage Label files with Model:
In Microsoft Dynamics AX 2012, label files, or ALD files, are part of models. A label file must be added to a model before the model can be installed. After a model has been installed, ALD files are pulled from the model store to the local of Application Object Server (AOS) instance when the AOS is started. When the AOS is shut down, the ALD files are pushed back to the model store.
ALD files from earlier versions of Microsoft Dynamics AX are not part of a model file. However, you can import these files into the model store from the Label Files section of the Application Object Tree (AOT). Use the Create from file shortcut command.
Note: The ALD file from an earlier version of Microsoft Dynamics AX must not be located in the application folder of AOS. Otherwise, you cannot import the file.
Working with label files across solutions
It is recommend that you use one label file per solution to simplify installation.
If you find that you require multiple label files, it is recommend that you create a single shared, cross-solution label file and package it as a model file. Then, when you install solutions, you must install two models: the solution itself and the label model.
If you want to ship additional languages, you can add the languages to the solution model, increment the model's version number, and then reimport the model.
Q) why do we use queries?
Ans:queries are user interactive, faster, compiles, reusable components
query, queryrun,querybuilddatasource,querybuildrange
if(qr.prompt())
Q) what is difference between temporary table and container???
Ans:when there are more than 50 columns then we go for temp tables and also there are some advantages using temp tables - we can create index, methods, fieldgroups
Q) How do u share tables across the companies????
Ans: Tablecollections and virtual companies
Q) when is configuration key used??
Ans:To enable and disable the features for all users [tables, fields, indexes, form controls, edt.enum...etc]
Security key for set of users or usergroups
- tables, forms, report, menuitems etc
RLS [Record level security]
Q) What are display methods?
Ans:Display methods will help you to show the data from some other table or hard-coded strings..these are not bound controls and we cannot use filter or sorting options
D365 Basics (Architeture, Models, Packages, Files)
The template project types are:
Dynamics AX Best Practice Rules – used for writing best practices checks for code and metadata
Dynamics AX Developer Tool Add-in
Dynamics AX Project – main project template type for containing customizations
Models, Packages, Files
KeyPoints
- Circular referencing is not allowed
- The concept of models is more or less the same as previous versions of AX 2012. In Dynamics 365 they are a design time concept that groups meta data and source files. Packages have have multiple models associated with Them
- Every model still exists within a single layer but that concept does not mean as much as in AX 2012 if the approach taken for development in D365 is extension. If the approach is approach is to overlay, which is the only development approach in previous version of AX, then layers become more important.
- The file structure is saved as XML , Each folder is a package with has sub folders of the models
- If you want to override a method, create a new class extending the base class and override the method. Inheritance (parent / child relationship and overriding) isn’t related to extensions.
- If you want to run some extra code on the beginning or the end of the method, you can also use events. You don’t need class extensions for this either.
- You can extend any Enum that is marked extensible (IsExtensible=True).
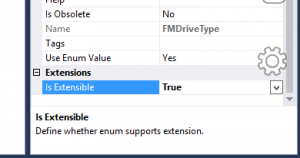
By extending an Enum, you can add new Enum values to it. It is important to keep the following in mind when dealing with extensible Enums:
- You cannot have X++ logic that depends on the integer value of Enum values (For example. If (Enum1.v1 > Enum1.v2) ... is not supported for extensible enums)
- When Enum values of extensible Enums are synchronized into the database:
- Integer values that belong to the baseline enum are deterministic, they come from the metadata.
- Integer values that are an extension are generated during the synchronization process and are not deterministic.
Packages
- It includes all of the models, binaries and additional pieces needed to deploy code.
- Similar concept to an AX2012 modelstore or in VS a solution.
- You can have multiple packages per installation.
- AX ships with several packages including:
- Application Suite: This is the package containing most of the application code and is the most likely to be overridden.
- Application Suite Form Adaptor
- Application Foundation
- Application Foundation Form Adaptor
- Application Platform
- Application Platform Form Adaptor
To create customization objects (overlayer objects) you have to:
Create a new model in the same package as the objects being customized.
This is because there will be a dependency between your customizations and the objects being customized.
The new model has to exist in a layer that you can access (usr, cus, or var).
Create a new model in the same package as the objects being customized.
This is because there will be a dependency between your customizations and the objects being customized.
The new model has to exist in a layer that you can access (usr, cus, or var).
AXPP Files
1. In the Solution Explorer, right-click on a project and choose Export project <ProjectName>
2. The project gets saved as a file with an extension of .axpp
a. The model the project is created in is saved in the axpp file, The model contains the layer info
b. The axpp file cannot be read with Notepad
3. To import the axpp containing the project in VS go to the Dynamics AX menu and choose Import project.
a. The project is created in the model (and layer) and saved in the axpp file.
b. If the model doesn’t exist in the imported environment, it will be generated.
4. Similar to 2012 and importing an xpo, in Dynamics 365 for Operations (AX7) you can do a compare between the contents of the axpp file and the current environment.
a. To compare objects during the import: When you select Import project a dialog opens asking you to select the location of the axpp file.
1)When you select Import project a dialog opens asking you to select the location of the axpp file.
2)Once you have selected the axpp file at the bottom of the dialog there is a Details section.
3)In the details section, you can see all of the objects to be imported.
4)When viewing the objects, right-click on one of them and choose Compare to bring up compare window.
Event Handlers
https://dynenterprisestrategies.com/news/2017/4/9/event-and-event-handlers-d365o
Naming Guidelines
https://docs.microsoft.com/en-us/dynamics365/unified-operations/dev-itpro/extensibility/naming-guidelines-extensions
Table Methods
[ExtensionOf(tableStr(InventTable))] final class MyInventTable_Extension { [DataEventHandler(tableStr(InventTable), DataEventType::Inserting)] public static void InventTable_onInserting(Common sender, DataEventArgs e) { InventTable inventTable = sender as InventTable; // Call the method as if it was defined directly on InventTable. inventTable.defaultMyInventLocationId(); } public void defaultMyInventLocationId() { // This would have partner specific logic to initialize the new field. this.MyInventLocationId = this.inventLocationId(); } }
Naming Guidelines
https://docs.microsoft.com/en-us/dynamics365/unified-operations/dev-itpro/extensibility/naming-guidelines-extensions
Table Methods
[ExtensionOf(tableStr(InventTable))] final class MyInventTable_Extension { [DataEventHandler(tableStr(InventTable), DataEventType::Inserting)] public static void InventTable_onInserting(Common sender, DataEventArgs e) { InventTable inventTable = sender as InventTable; // Call the method as if it was defined directly on InventTable. inventTable.defaultMyInventLocationId(); } public void defaultMyInventLocationId() { // This would have partner specific logic to initialize the new field. this.MyInventLocationId = this.inventLocationId(); } }
You can now add new methods to the augmentation class. These methods will then appear in IntelliSense for variables of the InventTable type, just as if they were defined directly on the table. This behavior applies to both static methods and instance methods.
There are a few rules for augmentation classes:
- They must be final.
- They must be suffixed by _Extension.
- They must be decorated with the [ExtensionOf()] attribute.
Note
In this example, the data event handling method is also defined on the augmentation class. In a real implementation, you might want to move the data event handling method into a separate class that contains the event handlers for the InventTable table.
Changes to the X++ language in Dynamics 365 for Operations
For the most part, the X++ language in Dynamics 365 for Operations is the same as it has always been. This is good news for all of us who have been active in the language for several years. Learning a new language is one thing we don’t need to worry about when moving to the latest version of the product. Having said that, here are some small language tweaks you might find interesting:
7 Language tweaks to X++
1. You can declare variables anywhere you want to. Variables don’t have to be declared before you use them.
2. In all previous versions, if you declared a variable in the classDeclaration you could not set it in the classDeclaration. Now you can set them as soon as you declare them.
3. You can use constants rather than macros. Macros are still supported but they appear to be well on their way out of the language.
4. The keyword var can be used when declaring variables. You don’t have to specify the exact type; the system will figure it out for you. This feels like lazy programming so you may or may not be interested in this change.
5. In try/catch statements you can use a finally block that will get executed regardless of whether an exception is thrown.
6. When referring to managed assemblies you can add a using statement to your X++ class. Once you have a using statement, you don’t have to use the full namespace in your X++ code to call the objects within the referenced assembly.
7. When building cross company statements, if you want to select from a subset of companies, you do not have to add the subset to a container. You can put the companies directly in the select statement like this:
select crossCompany : ([‘company1’] + [‘company2]) SalesTable;
Subscribe to:
Posts (Atom)
D365 Extensions: Extend the validateField Method on a Table
In this post I will going to show you, how to extend the validateField method on a table. As example I used the SalesLine, where I will ...

-
Hi All, There is a method named orig() in AX which refers to the last saved state of the current table buffer. The SalesTable form...
-
In this post I will going to show you, how to extend the validateField method on a table. As example I used the SalesLine, where I will ...
-
Hi All, We all know about “View Details” Option in our Ax 2012 Forms and Tables. Here I wrote one sample for how to get that functional...